1. Localizing Error Messages
이 레슨에서는 CAP 애플리케이션에서 오류 메시지를 현지화하는 방법을 배웁니다.
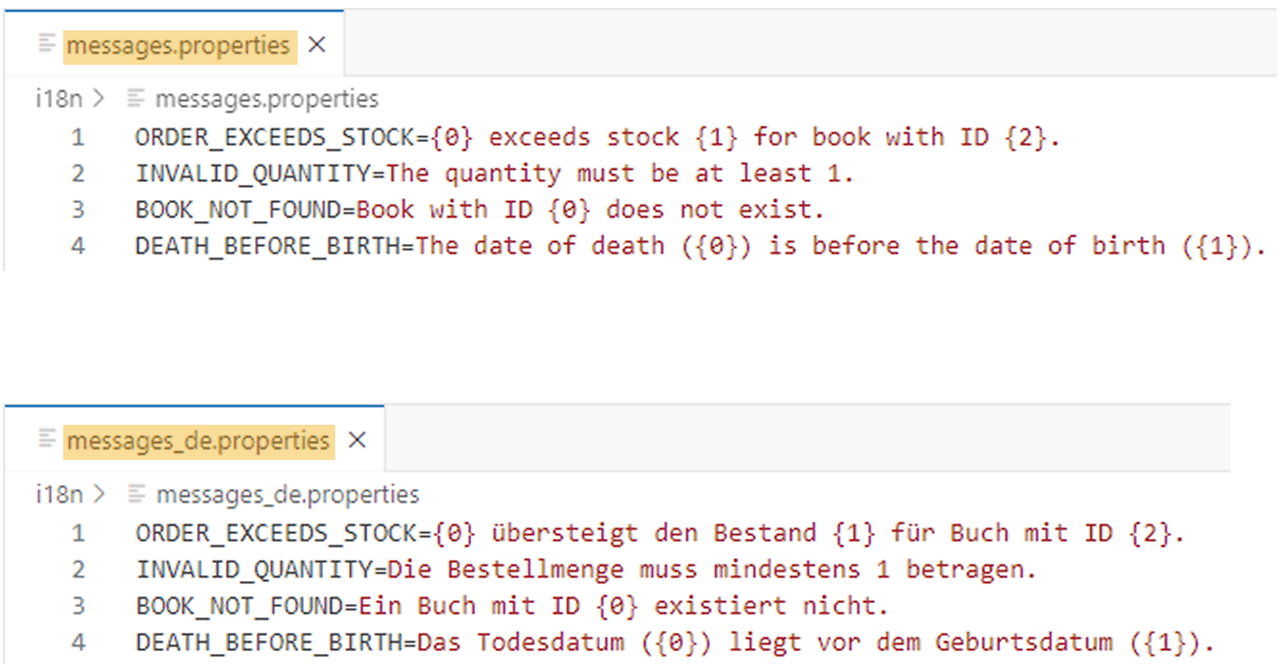
텍스트 번들 파일
- 오류 메시지를 하드코딩하는 대신 별도의 텍스트 번들 파일에 키-값 쌍으로 유지관리합니다.
- 애플리케이션 코드는 키를 사용하여 해당 텍스트에 접근합니다.
- 여러 언어를 지원하기 위해 동일한 키에 대해 번역된 텍스트를 제공하는 여러 파일을 유지관리합니다.
번들 파일 생성 절차
- _i18n, i18n 또는 assets/i18n 폴더에 messages.properties 파일을 생성합니다.
- 키-값 쌍으로 텍스트를 유지관리합니다 (보통 영어로).
- 추가 언어 지원을 위해 messages<_languageCode><_countryCode>.properties 형식의 파일을 생성합니다.
폴백 체인
- 요청된 언어의 파일이 없거나 키가 없는 경우 messages.properties로 폴백합니다.
- 그래도 없으면 키 이름을 텍스트로 사용합니다.
현지화된 메시지 접근
admin-service.js
validateLifeData(req) {
const { dateOfBirth, dateOfDeath } = req.data;
if (!dateOfBirth || !dateOfDeath) {
return;
}
const birth = new Date(dateOfBirth);
const dead = new Date(dateOfDeath);
if (dead < birth) {
req.error('DEATH_BEFORE_BIRTH', [dateOfDeath, dateOfBirth]);
}
}
- req.error() 또는 req.warn() 메서드를 사용하여 접근합니다.
- 실제 메시지 대신 키를 전달합니다.
- 플레이스홀더 값을 배열로 전달할 수 있습니다.
테스트
- URL 파라미터 sap-locale을 사용하여 다른 언어를 테스트할 수 있습니다.
- Accept-Language 헤더를 사용할 수도 있습니다.
실습 단계
- i18n 폴더 생성
- messages.properties 파일 생성 및 영어 텍스트 추가
- messages_de.properties 파일 생성 및 독일어 텍스트 추가
- admin-service.js와 cat-service.js 파일에서 현지화된 텍스트 사용
- 서버 시작 및 테스트 수행
이를 통해 CAP 애플리케이션에서 다국어 오류 메시지를 효과적으로 관리하고 제공할 수 있습니다.
2. Controlling Access to Data
CAP 애플리케이션에서 데이터 접근을 제어하기 위해 인증(Authentication)과 권한 부여(Authorization)를 사용하는 방법을 설명합니다. 이 과정에서는 주석(annotation)을 사용하여 세부적인 접근 제어를 설정합니다.
인증과 권한 부여의 개념
- 인증(Authentication): 사용자의 신원을 확인하는 과정.
- 권한 부여(Authorization): 인증된 사용자에게 특정 작업을 수행할 수 있는 권한을 부여하거나 제한하는 과정.
데이터 접근 제어를 위한 주석 사용
@readonly와 @requires
- @readonly: 엔터티를 읽기 전용으로 설정.
- @requires: 특정 역할(예: authenticated-user)을 요구하여 작업 수행을 제한.
예제: cat-service-auth.cds
using {
CatalogService.Authors,
CatalogService.Books,
CatalogService.submitOrder
} from './cat-service';
annotate Authors with @readonly;
annotate Books with @readonly;
annotate submitOrder with @(requires: 'authenticated-user');
- Authors와 Books 엔터티는 모든 사용자에게 읽기 전용으로 설정.
- submitOrder 액션은 인증된 사용자만 실행 가능.
@restrict
- 더 복잡한 조건으로 접근을 제한할 때 사용.
- 속성:
- grant: 허용할 이벤트(예: READ, CREATE, UPDATE, DELETE).
- to: 허용할 사용자 역할(예: admin).
- where: 필터 조건(선택 사항).
예제: admin-service-auth.cds
using { AdminService.Authors, AdminService.Books } from './admin-service';
annotate Authors with @(requires: 'admin');
annotate Books with @(restrict: [
{ grant: ['DELETE'], to: 'admin', where: 'stock = 0' },
{ grant: ['READ', 'CREATE', 'UPDATE'], to: 'admin' }
]);
- Authors: 관리자(admin)만 접근 가능.
- Books:
- 삭제(DELETE)는 재고(stock)가 0인 경우에만 관리자에게 허용.
- 읽기, 생성, 업데이트는 관리자에게만 허용.
인증 전략
개발 환경에서의 인증(Mocked Authentication)
- 기본적으로 모의(mocked) 인증 전략이 사용됩니다.
- package.json 파일에서 사용자와 역할을 정의 가능.
예제: package.json
"cds": {
"requires": {
"auth": {
"kind": "mocked",
"users": {
"adminuser": {
"password": "abcd1234",
"roles": ["admin"]
},
"catuser": {
"password": "abcd1234"
}
}
}
}
}
- adminuser: 패스워드 abcd1234, 역할은 admin.
- catuser: 패스워드만 있고 별도의 역할 없음.
프로덕션 환경에서의 인증
- 기본적으로 JWT(JSON Web Token)를 사용하여 인증.
- UAA(User Account and Authentication) 서비스와 통합하여 역할 및 사용자 속성을 제공.
테스트
Mocked 인증 테스트
요청에 Basic 인증 헤더를 추가하여 테스트:
GET <service_url>/Books
Authorization: Basic catuser:abcd1234
테스트 시나리오
- 인증 없이 엔터티 읽기 요청 → 허용됨.
- 인증 없이 데이터 생성 요청 → 거부됨 (@readonly 적용).
- 인증 없이 submitOrder 호출 → 거부됨 (@requires 적용).
- 관리자(adminuser)로 엔터티 읽기/생성/삭제 요청 → 조건에 따라 허용 또는 거부.
3. Quiz
1. Which of the following names can you choose for the folder where you store your text bundles if you use CAP's default configuration?
보기:
- messages
- i18n
- _i18n
- bundles
- resources/texts
- assets/i18n
정답: i18n, _i18n, assets/i18n
설명: CAP의 기본 설정에서 텍스트 번들을 저장하기 위해 사용할 수 있는 폴더 이름은 _i18n, i18n, assets/i18n입니다. 이 폴더들은 cds.i18n.folders 속성에서 기본값으로 지정됩니다.
2. Which of the following are valid annotations that can be used in CAP to control access to resources?
보기:
- @readonly
- @requires
- @updateonly
- @insertonly
- @restrict
- @deleteonly
- @check
정답: @readonly, @requires, @insertonly, @restrict
설명:
- @readonly: 엔터티를 읽기 전용으로 설정합니다.
- @requires: 특정 역할을 요구하여 접근을 제한합니다.
- @insertonly: 엔터티를 생성 전용으로 설정합니다.
- @restrict: 더 복잡한 조건으로 접근을 제한할 수 있습니다.
나머지 옵션(@updateonly, @deleteonly, @check)은 CAP에서 유효한 주석이 아닙니다.
출처: learning.sap.com